APRIL 16, 2020
Recent Blog
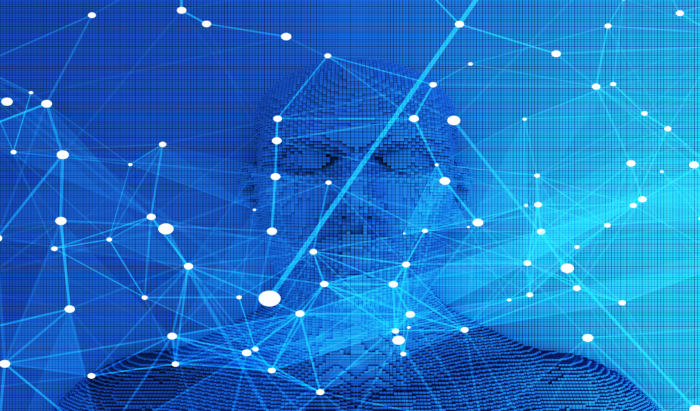
NOVEMBER 26, 2020
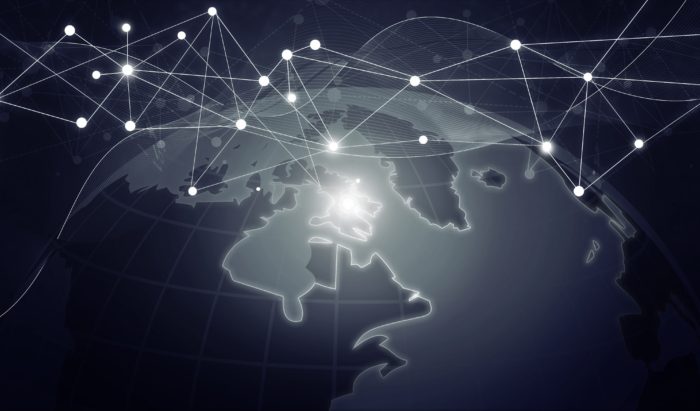
NOVEMBER 4, 2020
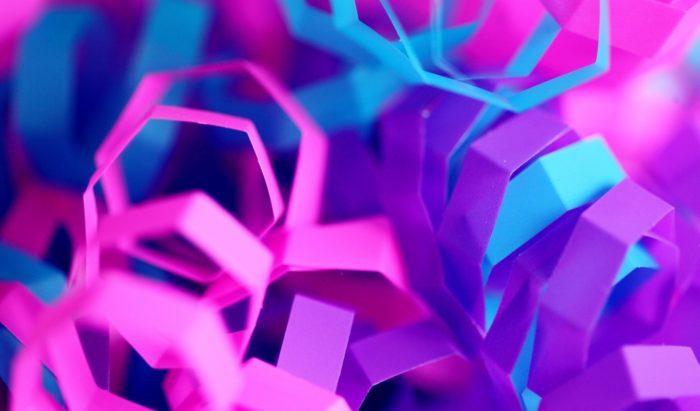
APRIL 16, 2020
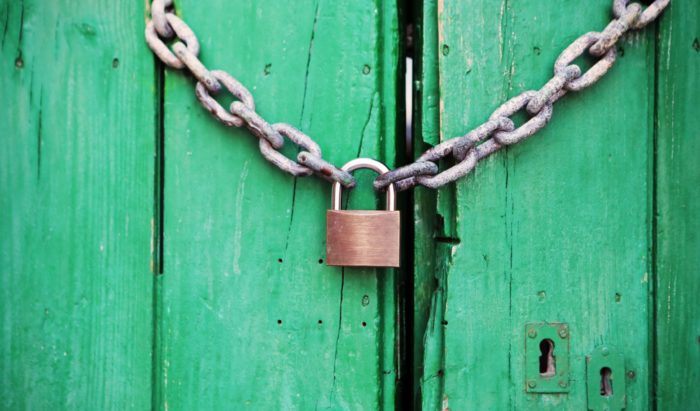
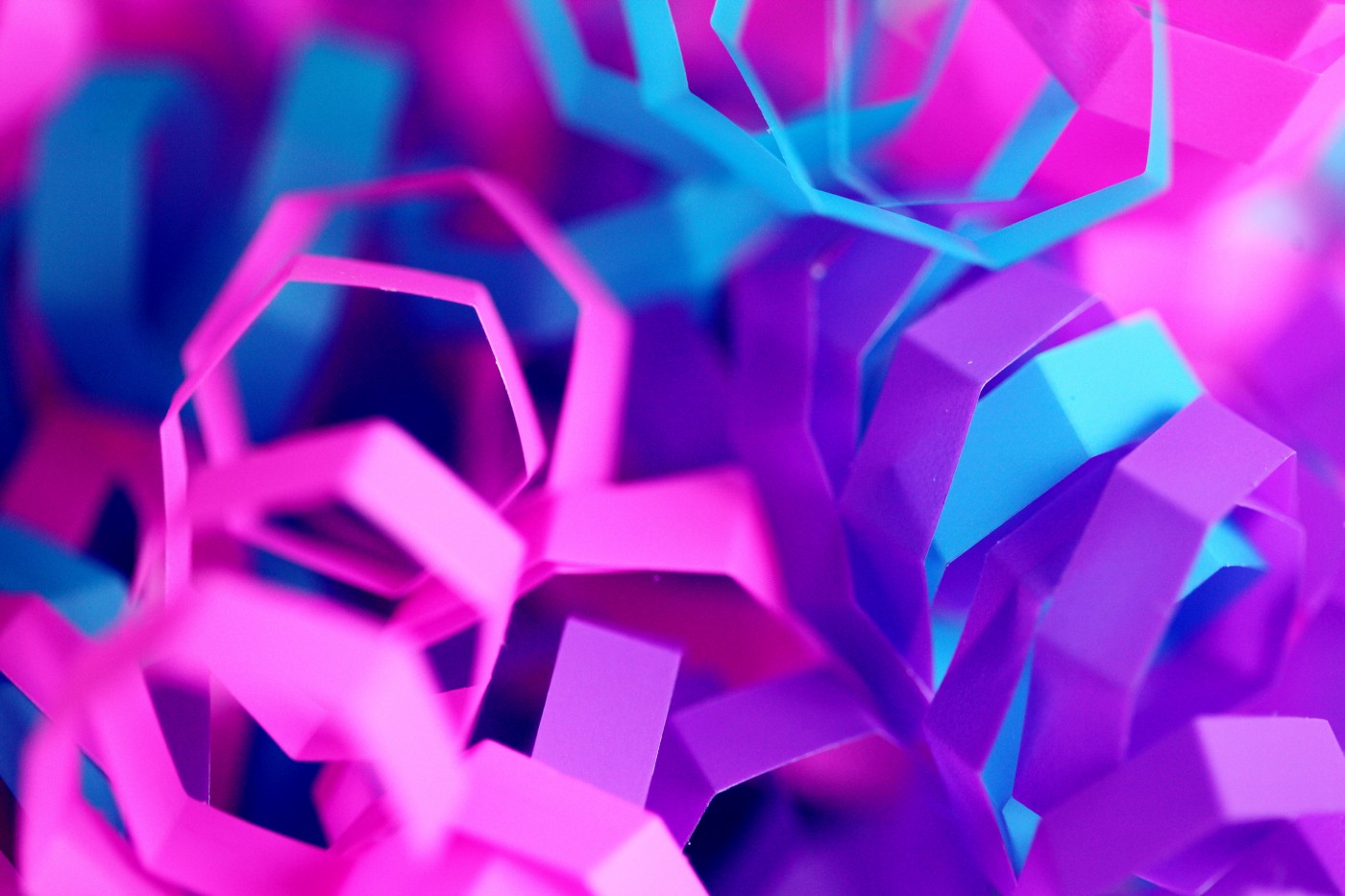
Wait, What is GraphQL?
Is it a new query language? A new type of database? Some other JavaScript black magic? Nope, none of the above.
in a nutshell, GraphQL is a Query Language for APIs that describes how to ask for data and is generally used to load data from a server to a client. Firstly, it doesn’t have anything to do with databases. It is not an alternative to SQL. It is basically used to send/receive data between client and server using a minimum number of HTTP requests. In order to achieve this, you have to create relations between the entities programmatically. That’s how it is called as a query language.
“Ask for what you need, get exactly that.”
Who’s using GraphQL?
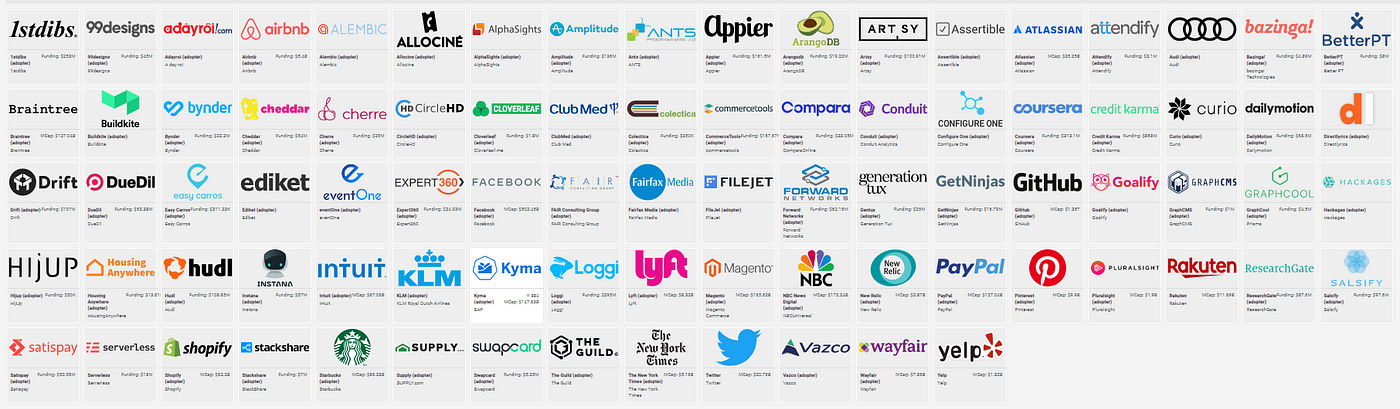
Organizations using GraphQL
Is GraphQL named after Graph? Yes.
“A small, miniscule speck of dust in an endless ocean of massive stars, trillions upon trillions of planets and energy. That is Universe Graph. Every object in the universe is interconnected to each other. We are an intrinsic part of it. Believe it or not, we are Star’s Stuff.”
In computer science, the Graph represents structure used to model pairwise relations between various objects(or nodes).
Understanding, using, and thinking in graphs makes us better programmers. At least that’s how we’re supposed to think. Graph-based APIs model the data in terms of nodes and edges (objects and relationships) and allows the client to interact with multiple nodes in a single request. With GraphQL, you model your business domain as a graph by defining a schema. within your schema, you define different types of nodes(data sources) and how they connect/relate to one another. That’s how it is called GraphQL.
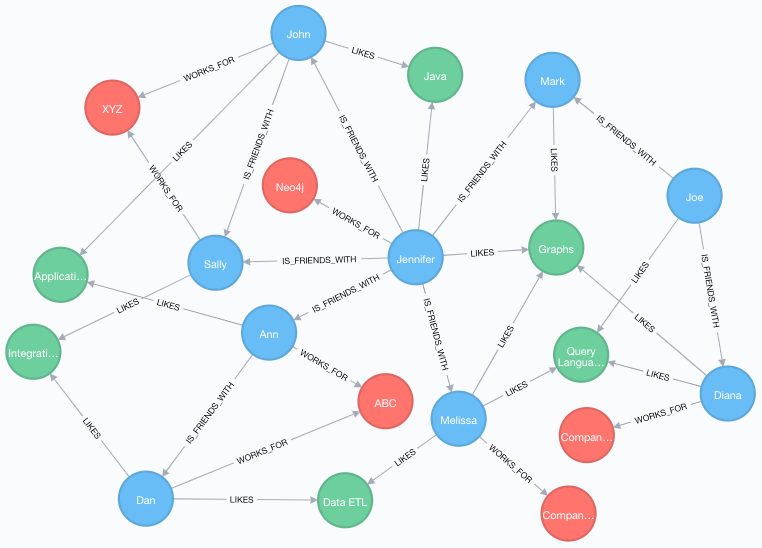
Nodes and Relations in Graph — Source
Why GraphQL is a Better REST API?
When you need to build an API, your mind will likely jump toREST, the standard for API creation. However, this is changing with the increase in GraphQL popularity. let’s see why GraphQL became popular within no time and why it is better than REST.
Resources Handling:
Now let’s say later down the line you wanted to build a simple note maker application that consists of creating, reading, updating and deleting notes operations. So, in the scenario of REST API, you would end up writing different routes for these operations right? well, that makes sense to the application for now right? So, it does mean that number of resource endpoints in your API greatly increases with the number of operations your Application. Guess what happens in the GraphQL world? You write a query to ask for what you want(create, read, update, delete), and you get back exactly what you asked for with a single endpoint. It doesn’t matter how big is our application, GraphQL can handle all your application operations with a single endpoint. How cool is that?
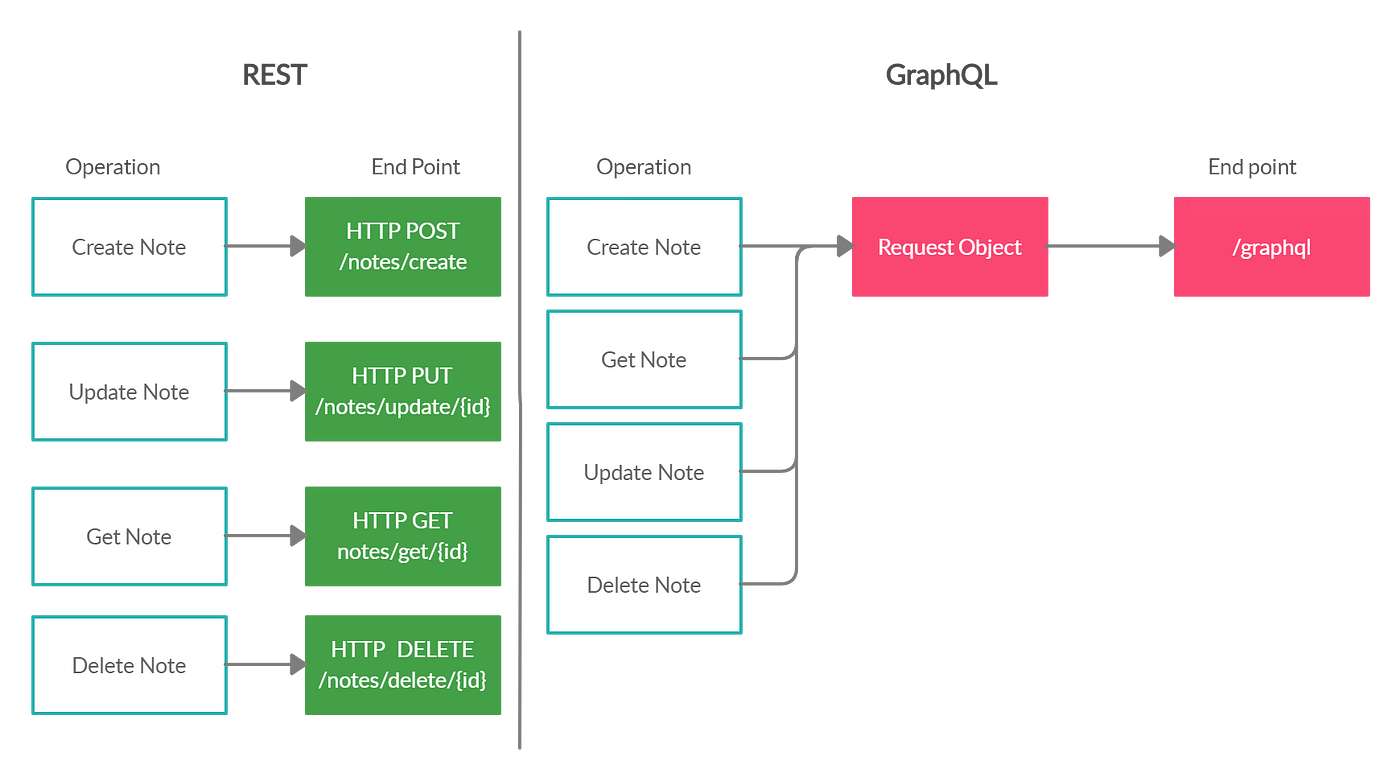
REST vs GraphQL Architecture
2. Data Fetching:
REST APIs are usually a collection of endpoints, where each endpoint represents a resource. so when a client needs data from multiple resources, it needs to perform multiple requests to API to get the data needed for. This Problem in REST API is called under-fetching — Under-fetching generally means that a specific endpoint doesn’t provide enough of the required information. The client will have to make additional requests to fetch everything it needs. let’s have a look at a basic social media application example of retrieving user details and his first 100 friends. with the REST API, you need to do multiple round trips on two endpoints to achieve this. let’s see how we can use GraphQL to solve this under-fetching problem.
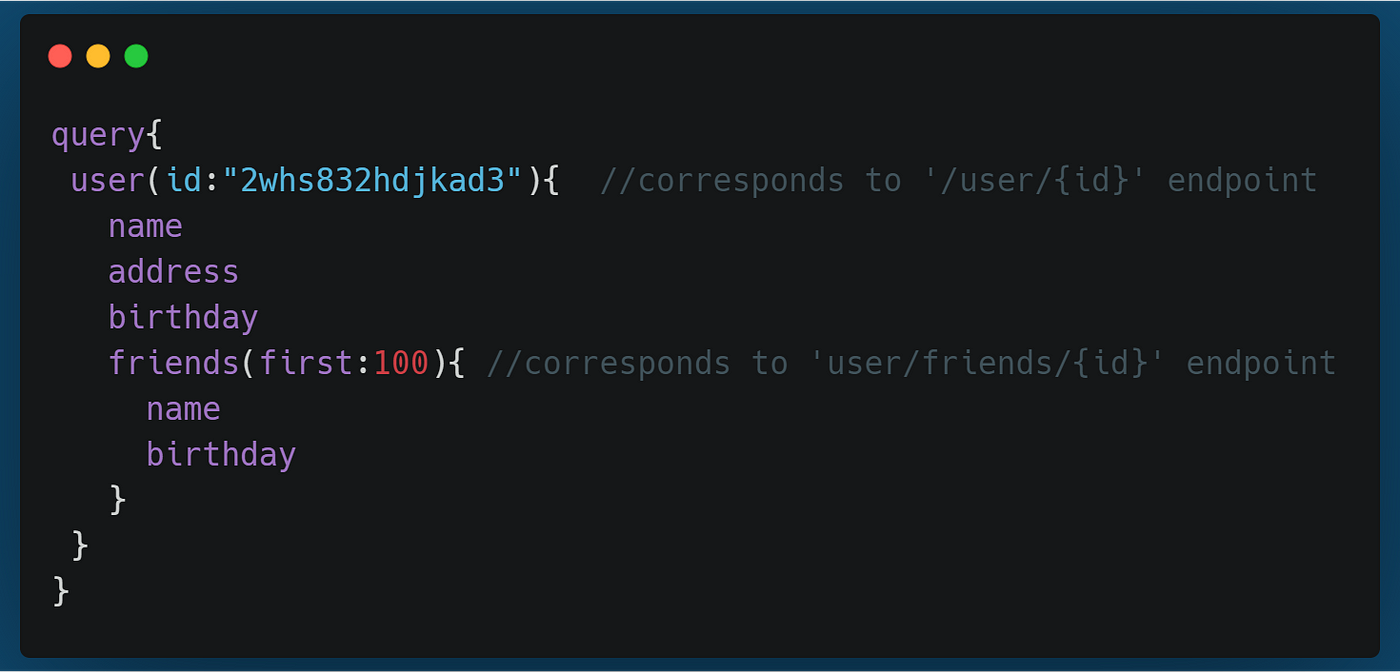
GraphQL query to solve an under-fetching issue in REST API with the single query in GraphQL, you will get whatever you want. nothing more or nothing less. nevertheless, you can also filter how many records you want which is yet complex to achieve with REST API.
Another problem we face with the REST API is over-fetching. — fetching excessive data that we don’t need. This REST implementation represents a huge waste bandwidth per request. for example, let’s have a look at a basic social media application that displays registered users on the home page of application just like facebook’s Add friends page. with REST API, when we call /users/allUsers endpoint, it will return a bunch of user records that are registered on the platform with the following fields of each user as an example.
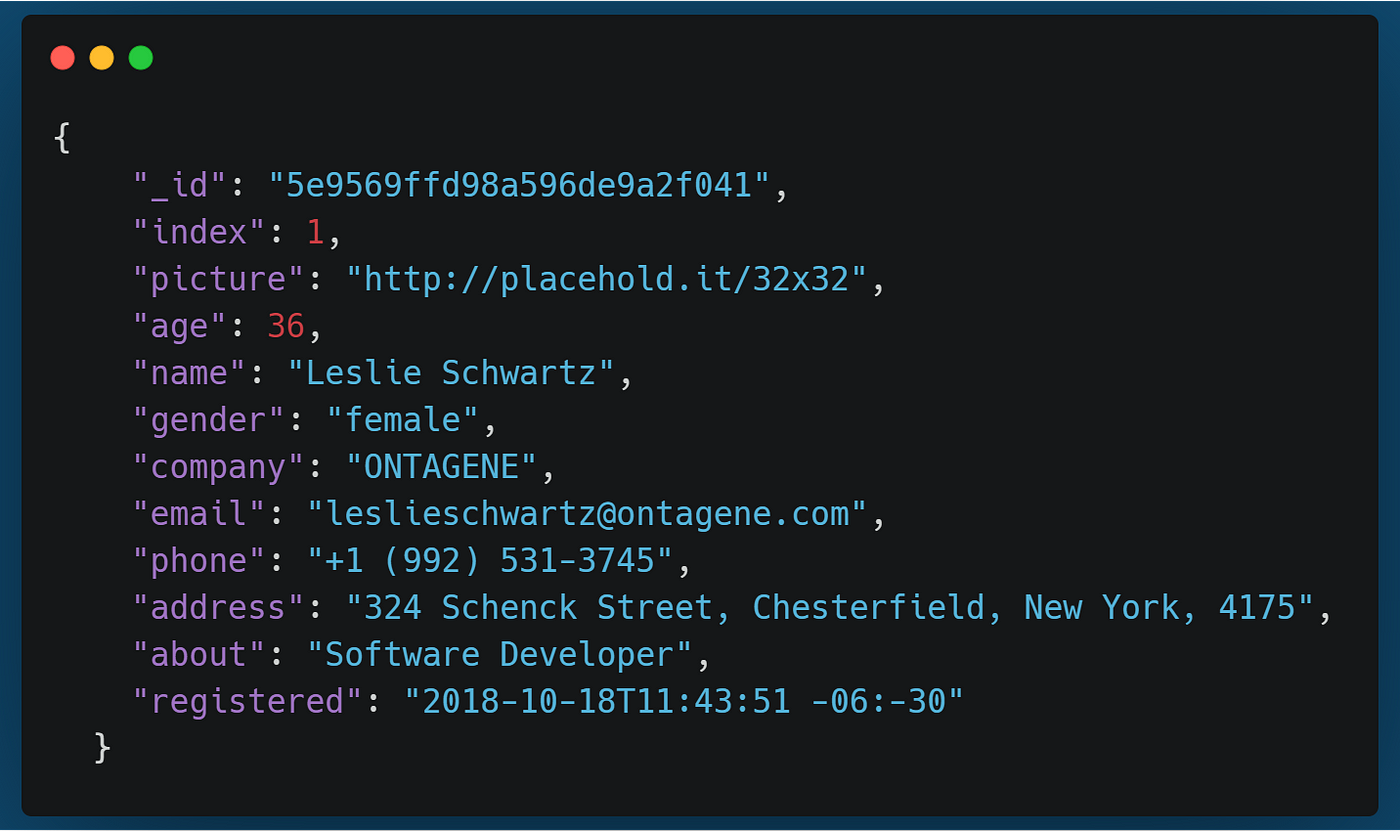
we might not use all these fields on the home page of the application to display users and also an excessive list of users (more than we required to display on the home page i.e. 20 users). So it is the waste bandwidth and network resources. With the GraphQL, we can fetch the fields that we need and list of records we’ve asked for with a simple query.
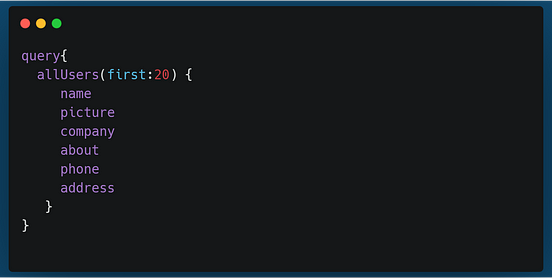
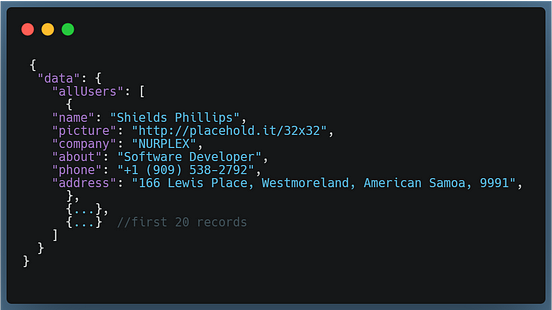
GraphQL — Fetching only what you asked for. Here in the query allUsers, we are specifying how many records we want and what fields does each record should return. Also, notice that the shape of the response matches exactly with the shape of the request. This way, we can ask for data in the form required rather than getting the default returned by the REST GET query.
3. API Versioning:
with REST, it’s common to offer multiple API versions. However, GraphQL eliminates the need for versioning by deprecating APIs on a field level. Aging fields can be later removed from the schema without impacting the existing queries. GraphQL enables that by creating a uniform API across the entire application that isn’t limited by a specific storage engine.
By using a single, evolving version, GraphQL APIs give apps continuous access to new features and encourage cleaner, more maintainable server code.
4. Debugging:
In REST, we simply check the HTTP headers for the status of the response, based on which we can determine what went wrong and how to handle it. Contrarily, if there is an error while processing GraphQL queries, the backend will provide a detailed error message including all the resolvers and referring to the exact query part at fault.
GraphQL error messages don’t have a particular standard, so you can choose — be it a stack trace, an application-specific error code, or just plain text.
5. API Documentation:
With REST we can use Swagger, RAML or other technologies to document our API and generate HTML documentation that our consumers can read without any need of interaction with the servers. The role of documentation with a REST API is to explain the individual endpoints, what function they perform, and the parameters a developer can pass to them. GraphQL comes with self-documenting capability. i.e. autogeneration of API documentation based on GraphQL schema. GraphQL keeps documentation in sync with API changes. As the GraphQL API is tightly coupled with code, once a field, type or query changes, so do the docs. This directly benefits developers, since they have to spend less time documenting an API.
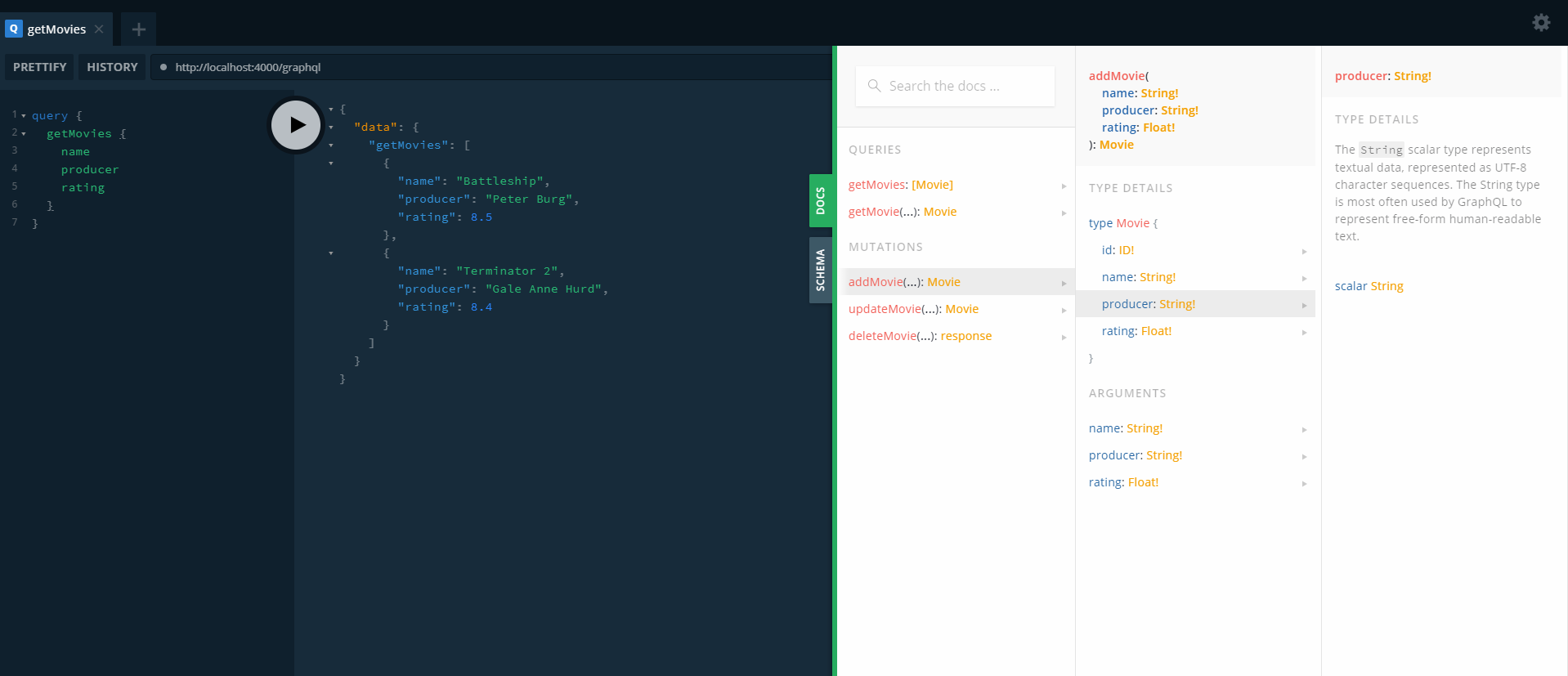
GraphQL API Documentation
6. A Powerful GraphQL Playground:
GraphQL comes with powerful Playground for better development workflows that make real-time requests to the schema, its features include interactive docs, an editor for GraphQL queries, tracing, sharing playgrounds, mutations, and subscriptions, validation, etc. Using the in-built GraphQL browser, you can experiment with the API and the data it exposes. It also has an auto-complete feature, If you’re not sure of the fields and relationships available, then start typing and the tool will auto-complete what’s available.
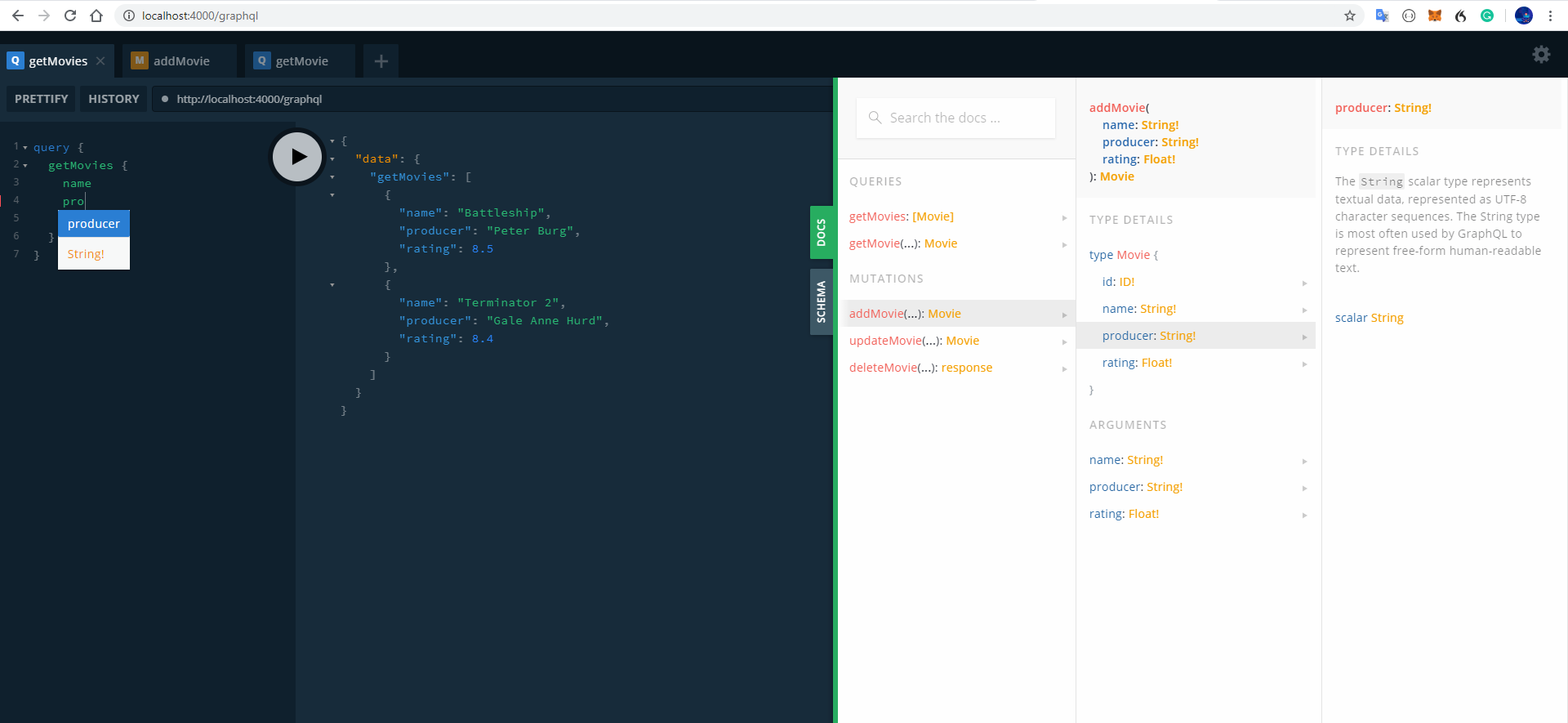
GraphQL IDE
Components of GraphQL Ecosystem:
Schema&Types:
GraphQL server uses a schema to describe the shape of your data graph. It defines the server’s API, allowing clients to know which operations can be performed by the server. The schema is written using the GraphQL schema language (also called schema definition language, SDL). The schema also specifies exactly which queries and mutations are available for clients to execute against your data graph. A schema defines a collection of types and the relationships between those types.
The GraphQL type system categorizes several custom types. common types are as follows:
a). normal object type:
The most basic components of a GraphQL schema are object types, which just represent a kind of object you can fetch from your service, and what fields it has.
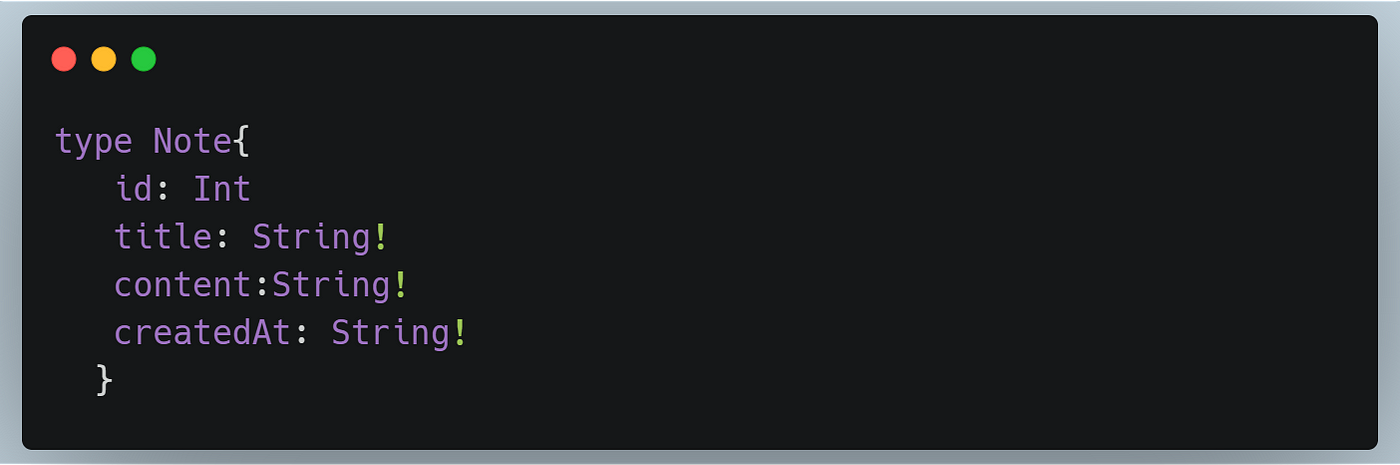
types in GraphQL are nullable by default. An exclamation point after a type (title:String! ) specifically designates that type as non-nullable(in other words ‘required’). So if it is null, it will throw an error instead of showing null.
b). root operation type
The root types are the query type, mutation type and subscription which are the three types of operations you can run requests from a GraphQL server. The query type is compulsory for any GraphQL schema, while mutation and subscription are optional.
a) Query type:
The Query type defines exactly which GraphQL queries (i.e., read-only operations) clients can execute against your data API.
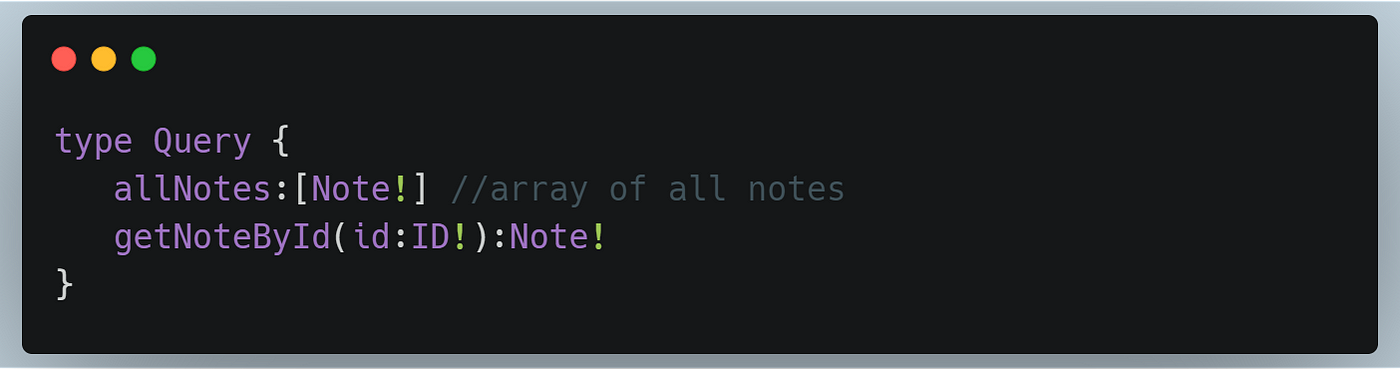
The aboveQuery type defines two available queries: allNotesand getNoteById. Each query returns a list of the corresponding type Note.
b) Mutation type:
If you have an API endpoint that alters data, like inserting data into a database or altering data already in a database, you should make this endpoint a Mutation rather than a Query. Simply, we can say, mutationis for CUD(Create, Update, Delete ) Operations.

This Mutation type defines all available mutations(i.e. write operations), addNote, updateNote, deleteNote. Each mutation accepts defined arguments and returns a newly created Note object. As you’d expect, this Note object conforms to the structure that we defined in our schema.
c) Subscriptions:
Ever wondered how Facebook is notifying(updating chatbox or notifications without making page refresh) when a new message/notification arrives? Subscriptions — Subscriptions are special features of GraphQL that allows a server to send data to its clients when a specific event happens. GraphQL subscription aims to help you build real-time functionality into your GraphQL applications. So from now on, when you get a new message or notification on Facebook, you might think “Ohh… That’s a GraphQL subscription…” right?. Have a look at below chat box example to understand how GraphQL subscriptions work in realtime.
.png)
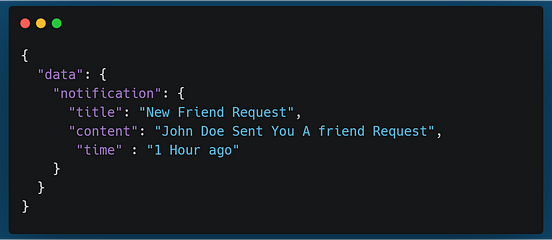
A Typical subscription type and response
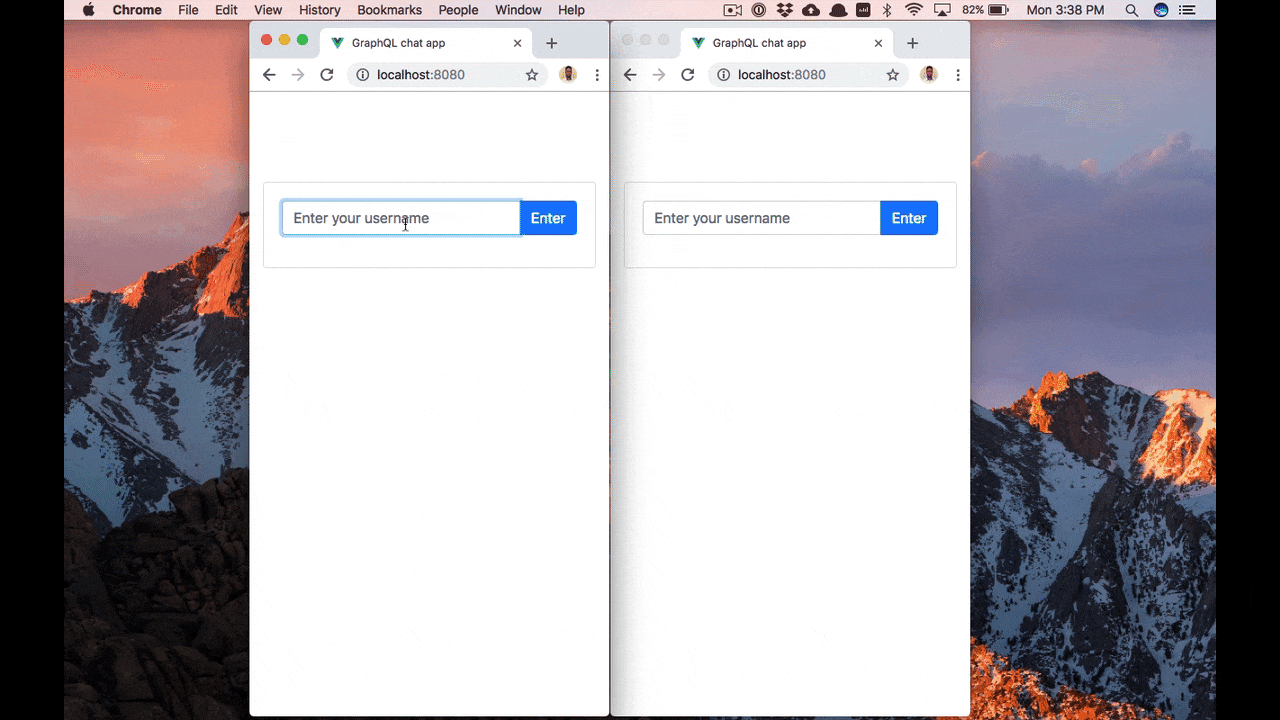
Source: https://pusher.com/tutorials/chat-graphql-subscriptions
2. Resolvers:
In order to respond to queries and mutations, a GraphQL schema needs to have resolver functions. A resolver is a function that is responsible for fetching data from the backend(Read Operations) and sending data to the backend(CUD Operations). resolver can contain as many functions (i.e queries and mutations) to get/send data from/to a backend database. This allows the GraphQL server to respond to different data sources from a single point of entry.
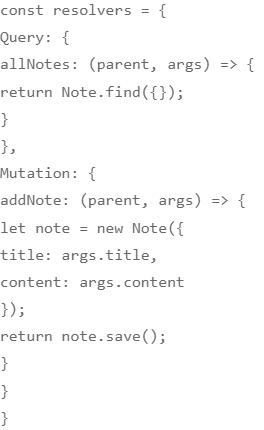
The above resolver for query allNotes returns all notes from the backend database. here Note.find() is a typical MongoDB query to return all documents in a particular collection. Similarly, resolver for mutation addNote takes the defined arguments and creates a new note document on the database. That’s how we will implement resolver functions to fetch/send data to the backend.
GraphQL API Architecture:
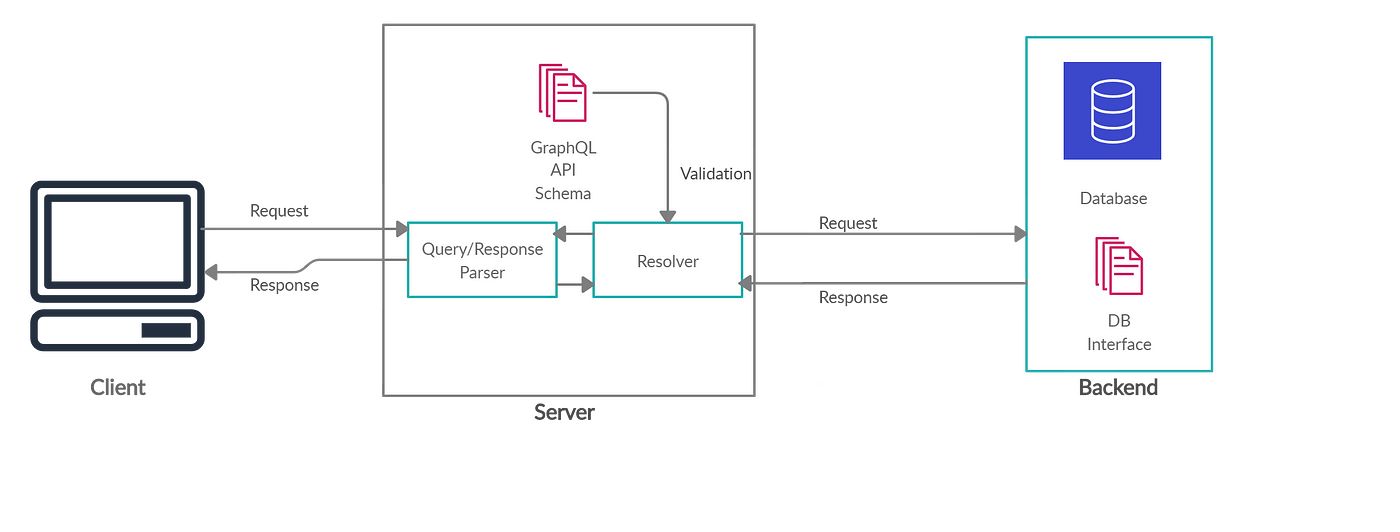
A Typical GraphQL API Architecture
Summary
- GraphQL is a Query Language for APIs that describes how to ask for data and is used to load data from a server to a client.
- GraphQL uses a single(or minimum number of) endpoints to send/receive data between client and server. it can handle all your application operations with a single endpoint.
- Using GraphQL we can solve over-fetching and under-fetching issues. because, with the GraphQL, the client gets only the data that it asked for and with a minimum number of requests.
- By using a single, evolving version, GraphQL APIs give apps continuous access to new features and encourage cleaner, more maintainable server code.
- Unlike REST showing HTTP error codes for an error, GraphQL will provide a detailed error message including all the resolvers and referring to the exact query/mutation part at fault.
- GraphQL comes with self-documenting capability. i.e. autogeneration of API documentation based on GraphQL schema. GraphQL keeps documentation in sync with API changes. So no need to re-document everything for API changes. Using the in-built GraphQL browser, you can experiment with the API and the data it exposes.
- The GraphQL schema is at the center of every GraphQL server. It defines the server’s API, allowing clients to know which operations can be performed by the server and what fields and types that every query returns.
- A GraphQL operation can either be a read or a write operation. GraphQL query is used to read or fetch the data while a mutation is used to write or alter the data. subscriptions are a GraphQL feature that allows a server to send data to its clients when a specific event happens.
- A resolver is a function that is responsible for fetching data from the backend(Read Operations) and sending data to the backend(CUD Operations).
We discussed here, what is GraphQL, Benefits of GraphQL over REST and various components of GraphQL Ecosystem and how they’re related to each other. I hope this article somehow helped you to get started with GraphQL. This is a three-part series of articles. In the next article, let’s dive deep into the components we discussed earlier and build a simple CRUD application using GraphQL, Mongo and Node. Are you guys interested? Follow us!!.